1. 什么是JavaScript
1.1 什么是javascirpt
- 运行在浏览器的脚本语言
- 实现在浏览器的动作,包括用户交互和数据处理
- 属于解析性语言,前端范畴里将其放在HTML里头
1.2 页面中的javascirpt代码
在<script>标签内
1 2 3 4 5 6 7 8 9
| <html> <head> </head> <body> <script> document.write('Javascript'); </script> </body> </html>
|
外部链接
1 2 3 4 5 6 7
| <html> <head> </head> <body> <script src=""></script> </body> </html>
|
1.3 桌面上的javascirpt
后端 – node.js,本地运行node环境
1 2 3 4 5 6 7 8
| var http = require('http'); http.createServer(function (req,res) { res.writeHead(200,{'Content-Type':'text/plain'}); res.end('Hello World\n'); }).listen(1337,'127.0.0.1'); console.log('Server runing at http://127.0.0.1:1337/');
|
1.4 事件响应代码
事件通常与函数结合使用,函数不会在事件发生前被执行。
DOM 0级事件。Javascript在HTML文档元素中注册不同事件处理程序。
1 2 3 4 5 6 7
| <!DOCTYPE html> <html> <head></head> <body> <p onclick="alert('Hello!')">Click here, please!</p> </body> </html>
|
DOM 2级事件。
1 2 3 4 5 6 7 8 9 10 11 12
| <!DOCTYPE html> <html> <head></head> <body> <p>Click here, please!</p> </body> <script> document.getElementsByTagName('p')[0].addEventListener('click',function () { alert('Hello!'); }) </script> </html>
|
1.5 变量
varible 变量
因为是松散类型,可以保存任何类型的数据,每个变量仅仅是一个用来保存值得占位符。
1 2 3 4 5 6 7 8
| * 定义变量 * var操作符,不要少 * 变量名 第一个字符不要是数字,可以由大小字母,数字,下划线组成,也不能是关键字 * ‘=’赋值符号 */ var a = 'hello'; document.write(a);
|
2. 计算与判断
2.1 基本计算
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| var a = 16; var bool = true; var str = 'abc'; document.write(str+a+'<br>'); document.write(a+2+'<br>'); document.write(-a+'<br>'); document.write(a*2/3+'<br>'); document.write(a%3+'<br>'); document.write((a+1)%3+'<br>'); document.write(a++); document.write(a);
|
2.2 比较
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| var a = 12; var b = 13; var c = '12'; var str1 = 'a'; var str2 = 'b'; console.log(a == b); console.log(a == c); console.log(a === b); console.log(a === c); console.log(a !== b); console.log(a <= b); console.log(a >= b); console.log(str1 > str2); console.log(str1 < str2);
|
3. 循环函数与数组
3.1 循环
3.1.1 while循环
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| var i=1000, j=0; while(i > 1) { i = i / 2; j++; } console.log(j); * 计算两个非负整数u和v的最大公约数gcd * 计算temp = u % v, u=v, v=temp; 如果v=0, gcd=u */ var getGcd = function (u,v) { var temp; while (v != 0) { temp = u % v; u = v; v = temp; } return u; } console.log(getGcd(42,21)); var a=1,b; while(a <= 5) { b = 1; while(b <= 3) { console.log(b); b++; } a++; }
|
3.1.2 do循环
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| var i = 10; do{ console.log(i); i--; }while(i > 0) var num = 12345; var reverse = 0; do{ var lastDigit = num % 10; reverse = (reverse * 10) + lastDigit; num = num / 10; }while(num > 0); console.log("That number reversed is " + reverse);
|
3.1.3 for循环
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| for (var i=0; i<5; i++) { console.log(i); } var amt = 74; for (var one = 0; one <= amt; ++one) { for (var five = 0; five <= amt / 5; ++five) { for (var ten = 0; ten <= amt / 10; ++ten) { for (var twenty = 0; twenty <= amt / 20; ++amt) { if (one + five * 5 + ten * 10 + twenty * 20 === amt) { console.log(one+" 张1元," +five+" 张5元,"+ten+" 张10元,"+twenty+" 张20元,组成"+amt+"元"); } } } } }
|
3.1.4 循环控制
- break 跳出循环
- continue 跳出当前循环步,进入循环下一轮,如无必要,变换方法不使用该语句
3.2 函数
3.2.1 函数
- 一段代码,起个名字,以后可调用
- 内置函数
1 2 3 4 5 6 7 8
| console.log(f1); console.log(f2); function f1() {} var f2 = function() {}
|
3.2.2 有返回值的函数
1 2 3 4 5
| var max = function (a,b) { return a>b ? a : b; } console.log(max(23,93));
|
3.2.3 函数变量
var f = new Function(“x”,”y”,”return x*y”);
等价于
var f = function (x,y) {return x*y;}
1 2 3 4 5 6 7 8 9 10
| var addBr = function(str) { return str+"<br>"; } var add = function (a,b) { return a+b; } var cal = function (fn,a,b) { return fn(a,b); } document.write(addBr(cal(add,5,6)));
|
3.2.4 变量空间
- 定义在函数外的变量,在全局可见
- 定义在函数内的变量,在函数内可见
- 全局和局部出现重名变量,采用局部变量
3.3 数组
3.3.1 数组
使用多个数据,每个数据将通过一个对应索引数字来访问,
1 2 3 4 5 6 7 8 9 10 11 12
| var a = new Array(); var b = new Array(5); var c = new Array(d1,d2,d3); var d = [d1,d2,d3]; var colors = ['red','blue','green']; colors[0] = 'black'; console.log(colors);
|
3.3.2 数组运算
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| var arr = [1,2,3]; console.log(arr.length); arr[5] = 5; console.log(arr.length); arr.length = 3; console.log(arr[5]); arr.length = 4; arr[arr.length] = 4; var colors = ['red','blue','green']; console.log(colors.toString()); console.log(colors.valueOf()); console.log(colors.join('||'));
|
3.3.3 数组其它操作
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54
| var arr1 = []; var count = arr1.push(1,2); console.log(count); console.log(arr1); var item = arr1.pop(); console.log(item); console.log(arr1); var arr2 = []; var count = arr2.unshift(1,2); console.log(count); console.log(arr2); var item = arr2.shift(); console.log(item); console.log(arr2); var arr3 = [0,1,5,10,15]; arr3.sort(); console.log(arr3); arr3.sort(function (a,b) { if (a<b) { return 1; } else if (a > b) { return -1; } else { return 0; } }); console.log(arr3); var arr4 = [1,2,3]; arr4.reverse(); console.log(arr4); var arr5 = arr4.concat(4); console.log(arr5); var arr6 = arr4.slice(1,4); console.log(arr6); var arr7 = [1,2,3,4]; arr7.splice(0,1); console.log(arr7); arr7.splice(3,0,5,6); console.log(arr7); arr7.splice(0,1,1); console.log(arr7);
|
4. 面向对象与事件处理
4.1 对象
4.1.1 对象
复合数据类型
将多个数据集中在一个变量,每个数据都起名字
属性集合,每个属性有名字和值
先有对象,再设置对象属性
1 2 3 4 5 6 7 8 9 10 11 12
| var obj = new Object(); var ciclr = {x:0,y:0,radius:2}; var book = new Object(); book.title = 'Hello World'; book.translator = 'mine'; book.chapter1 = new Object(); book.chapter1.title = 'Welcome'; book.chapter2 = {} delete book.chapter1; book.chapter2 = null;
|
4.1.2 遍历对象属性
1 2 3 4 5 6 7 8
| var obj = {} obj.name = 'Tom'; obj.age = 23; obj.salary = 400; for (var i in obj) { console.log(i + " : " + obj[i]); }
|
4.1.3 构造函数
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| * 不直接制造对象 * 通过this定义成员 * 没有return */ var Rect = function (w,h) { this.width = w; this.height = h; this.area = function () { return this.width * this.height; } } var r = new Rect(5,10); console.log(r.area);
|
4.1.4 对象原型
对象的prototype属性指定它的原型对象,可以用.运算符直接读它的原型对象的属性。
当写这个属性时候,才在它的内部产生实际的属性。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| var Person = function () {} Person.prototype.name = 'Nicholas'; Person.prototype.age = 29; Person.prototype.job = "Engineer"; Person.prototype.friends = ['Court']; Person.prototype.say = function () { console.log(this.name); } var person1 = new Person(); person1.say(); var person2 = new Person(); person2.say(); console.log(person1.say === person2.say); var person3 = new Person(); person3.name = "Greg"; console.log(person3.name); console.log(person1.name); delete person3.name; console.log(person3.name); person1.friends.push("Mike"); console.log(person1.friends); console.log(person2.friends);
|
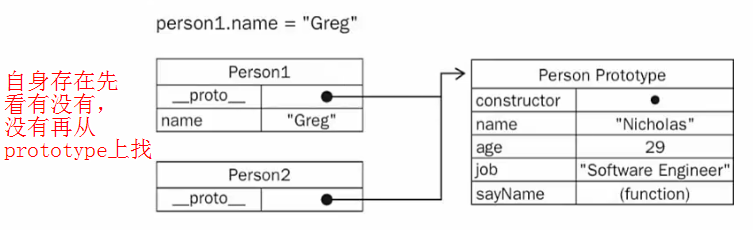
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| var Person = function (name,age,job) { this.name = name; this.age = age; this.job = job; this.friends = ['Court']; } Person.prototype = { constructor: Person, say: function () { console.log(this.name); } } var person1 = new Person('Nicholas',29,'Teacher'); var person2 = new Person('Greg',27,"Doctor"); person1.friends.push("Mike"); console.log(person1.friends); console.log(person2.friends); console.log(person1.friends === person2.friends);
|
4.2 事件
4.2.1 浏览器中的js
全局对象
浏览器的全局对象 – window
所有全局变量是window的成员
1 2 3
| > var str = 'hello' > console.log(window.str); >
|
document属性
表示浏览器窗口中的HTML页面
document.write() – 将内容写入页面
页面中的元素是document的成员
1 2 3 4
| > for (var i in document) { > console.log(i); > } >
|
HTML中的javascript
<script>标记中
src属性或者archive指定地址
标记的事件处理器中
4.2.2 事件处理器
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| <!DOCTYPE html> <html> <head> <title>Event</title> </head> <body onLoad='console.log("body load");' onUnload='console.log("body unload")'> <p onMouseOver='status="Print your name";' onMouseOut='status="";'> Mouse over here! You can click. </p> <p onMouseOver='alert("Hi");'></p> <script> var p1 = document.getElementsByTagName('p')[0], p2 = document.getElementsByTagName('p')[1]; p1.onclick = function () { if (confirm('Do you want to print your name?')) { var name = prompt("What is your name?","Bill"); p2.innerHTML = "Hello, " + name; } else { return } } </script> </body> </html>
|
4.2.3 定时器事件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| <!DOCTYPE html> <html> <head> <meta charset='utf-8'> <title>定时器</title> <script> var count = 10; function update() { if (count > 0) { status = count--; } } </script> </head> <body onLoad='setInterval("update()",1000);'> </body> </html>
|